A basic explanation of how the framework works is provided below. For a complete description of the design decisions when implementing the framework, see Implementation Details.
Programming the Agent
Embedded-BDI implements the agent reasoning cycle and necessary libraries to support AgentSpeak syntax. Therefore, users can focus on programming the agent behavior. Three files are required to program and compile the agent:
data/agentspeak.asl
: defines the agent behavior, coded in AgentSpeak;data/functions.h
: includes the belief update and action functions;agent.config
: specifies the size of the following internal data structures of the agent: event base, intention base, and intention stack.
Agent Behavior
Agent behavior can be described in the data/agentspeak.asl
file using AgentSpeak syntax. Be aware of the limitations of the framework, notably the lack of support for predicates. Details about the supported syntax for this file and agent features can be checked on the Supported Features page.
!start.
+!start <- +happy.
+happy <- !!hello.
+!hello <- say_hello.
Belief update and action functions
Because hardware used in embedded systems is heterogeneous and I/O interfaces can vary, users must provide the necessary functions to update beliefs and act in the environment. These functions should be provided in the data/functions.h
file, and must follow specific syntax: the update_
prefix must be used to associate the update function with its corresponding belief, and the action_
prefix shall be used to link the function with its corresponding body instruction. Note that:
- Both function types must respect its return type (
bool
) and arguments received (bool var
, forupdate_
functions); - While update functions are optional for beliefs, all actions should have a corresponding function.
#ifndef FUNCTIONS_H_
#define FUNCTIONS_H_
#include <iostream>
bool action_say_hello()
{
std::cout << "Hello world!" << std::endl;
std::cout << "I am an agent and I will keep running until " <<
"I am terminated" << std::endl;
return true;
}
bool update_sunny(bool var)
{
return true;
}
#endif /* FUNCTIONS_H_ */
Agent Configuration
Lastly, because of the limited resources available in embedded systems, memory usage must be pre-allocated and strictly managed for the internal data structures used by the agent. Hence, it is necessary to configure the size of the event and intention queues and the size of the intention stack. This can be done through the agent.config
file.
EVENT_BASE_SIZE=5
INTENTION_BASE_SIZE=5
INTENTION_STACK_SIZE=5
Compile agent executable
Once the three files are placed on their corresponding locations (data/functions.h
, data/agentspeak.asl
, and agent.config
), the agent executable can be compiled. The build process is split into two phases:
- AgentSpeak translation: first, the
data/agentspeak.asl
file is translated from the AgentSpeak language to a C++ header file (src/config/configuration.h
). - Agent compilation: once the agent code is translated to a corresponding C++ header file, the agent can be compiled using the Embedded-BDI library and the files provided by the user.
This process is illustrated by the image below:
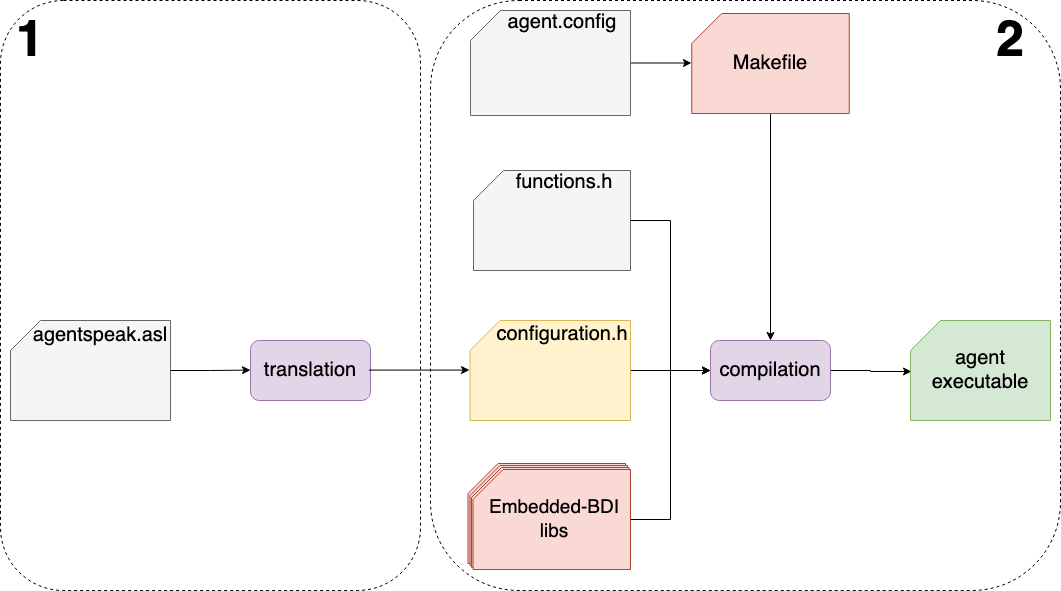
Instructions to build and run the agent are available here.